poj 1459 -- Power Network
Time Limit: 2000MS | Memory Limit: 32768K | |
Total Submissions: 22517 | Accepted: 11800 |
Description
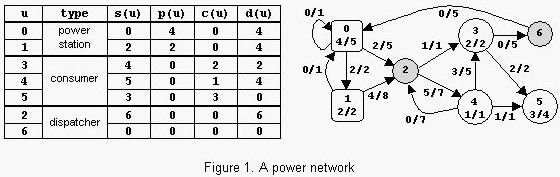
An example is in figure 1. The label x/y of power station u shows that p(u)=x and pmax(u)=y. The label x/y of consumer u shows that c(u)=x and cmax(u)=y. The label x/y of power transport line (u,v) shows that l(u,v)=x and lmax(u,v)=y. The power consumed is Con=6. Notice that there are other possible states of the network but the value of Con cannot exceed 6.
Input
Output
Sample Input
2 1 1 2 (0,1)20 (1,0)10 (0)15 (1)20 7 2 3 13 (0,0)1 (0,1)2 (0,2)5 (1,0)1 (1,2)8 (2,3)1 (2,4)7 (3,5)2 (3,6)5 (4,2)7 (4,3)5 (4,5)1 (6,0)5 (0)5 (1)2 (3)2 (4)1 (5)4
Sample Output
15 6
Hint

1 /*====================================================================== 2 * Author : kevin 3 * Filename : PowerNetwork.cpp 4 * Creat time : 2014-07-16 09:41 5 * Description : 6 ========================================================================*/ 7 #include <iostream> 8 #include <algorithm> 9 #include <cstdio> 10 #include <cstring> 11 #include <queue> 12 #include <cmath> 13 #define clr(a,b) memset(a,b,sizeof(a)) 14 #define M 105 15 #define INF 0x7f7f7f7f 16 using namespace std; 17 struct Edge{ 18 int from,to,cap,flow; 19 }; 20 int n,np,nc,m,supers,supert; 21 vector<Edge> edges; 22 vector<int> G[M]; 23 bool vis[M]; 24 int d[M],cur[M]; 25 bool BFS() 26 { 27 clr(vis,0); 28 queue<int>Q; 29 Q.push(supers); 30 d[supers] = 0; 31 vis[supers] = 1; 32 while(!Q.empty()){ 33 int x = Q.front(); Q.pop(); 34 int len = G[x].size(); 35 for(int i = 0; i < len; i++){ 36 Edge& e = edges[G[x][i]]; 37 if(!vis[e.to] && e.cap > e.flow){ 38 vis[e.to] = 1; 39 d[e.to] = d[x]+1; 40 Q.push(e.to); 41 } 42 } 43 } 44 return vis[supert]; 45 } 46 int DFS(int x,int a) 47 { 48 if(x == supert || a == 0) return a; 49 int flow = 0,f; 50 int len = G[x].size(); 51 for(int& i = cur[x]; i < len; i++){ 52 Edge& e = edges[G[x][i]]; 53 if(d[x] + 1 == d[e.to] && (f = DFS(e.to,min(a,e.cap-e.flow))) > 0){ 54 e.flow += f; 55 edges[G[x][i]^1].flow -= f; 56 flow += f; 57 a -= f; 58 if(a == 0) break; 59 } 60 } 61 return flow; 62 } 63 void AddEdge(int from,int to,int cap) 64 { 65 edges.push_back((Edge) {from,to,cap,0}); 66 edges.push_back((Edge) {to,from,0,0}); 67 int m = edges.size(); 68 G[from].push_back(m-2); 69 G[to].push_back(m-1); 70 } 71 int Dinic(int s,int t) 72 { 73 int flow = 0; 74 while(BFS()){ 75 clr(cur,0); 76 flow += DFS(s,INF); 77 } 78 return flow; 79 } 80 int main(int argc,char *argv[]) 81 { 82 int u,v,cap; 83 while(scanf("%d%d%d%d",&n,&np,&nc,&m)!=EOF){ 84 char str[10]; 85 for(int i = 0; i < m; i++){ 86 scanf("%s",str); 87 sscanf(str,"(%d,%d)%d",&u,&v,&cap); 88 AddEdge(u,v,cap); 89 } 90 supers = n; 91 int num; 92 for(int i = 0; i < np; i++){ 93 scanf("%s",str); 94 sscanf(str,"(%d)%d",&num,&cap); 95 AddEdge(supers,num,cap); 96 } 97 supert = n+1; 98 for(int i = 0; i < nc; i++){ 99 scanf("%s",str); 100 sscanf(str,"(%d)%d",&num,&cap); 101 AddEdge(num,supert,cap); 102 } 103 int ans = Dinic(supers,supert); 104 printf("%d\n",ans); 105 edges.clear(); 106 for(int i = 0; i < M; i++) 107 G[i].clear(); 108 } 109 return 0; 110 }
郑重声明:本站内容如果来自互联网及其他传播媒体,其版权均属原媒体及文章作者所有。转载目的在于传递更多信息及用于网络分享,并不代表本站赞同其观点和对其真实性负责,也不构成任何其他建议。